How to create and verify ECC signatures using the Elliptic Java library
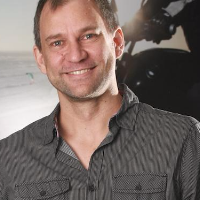
Kobus Grobler
The Java version of the Elliptic ECC Library is fairly straightforward to use. Here we demonstrate how to create and verify ECC signatures using the library.
First, you need to initialize the type of elliptic curve you are using:
// Initialize the optimal normal basis math library
ONB onb = new ONB();
// ECC curve on which we operate
ECurve crv = new ECurve();
onb.one(crv.a2);
onb.one(crv.a6);
crv.form = 1;
This will setup a curve with a2 and a6 = 1 of the form 1. The default number of bits is 113.
Then create the ECC parameters (with previously generated points on the curve). This is a Koblitz curve:
// Pre-generated ECC Parameters for this test
EPoint G = new EPoint(new BigInteger("5262620910903524821739262082116001"),
new BigInteger("1590460219736397884499739636162152"));
BigInteger koblitzPrimeOrder = new BigInteger("5192296858534827627896703833467507");
EParam eccParams = new EParam(crv,koblitzPrimeOrder,new BigInteger("2"),G);
Create a key pair:
// Random ECC Key pair used for signing data
EKeyPair keyPair = elliptic.genRandomPair(eccParams);
Then sign the data:
// Some data to sign
byte data[] = new byte[64];
for (int i = 0; i < data.length; i++) {
data[i] = (byte)i;
}
Elliptic elliptic = new Elliptic(onb);
// sign the data with the private key.
ESignature signature = elliptic.sign(eccParams,keyPair.priv,data);
At a later stage you can verify the signature generated over the data above using the verify method:
// verify the signature with the public key.
boolean verified = elliptic.verify(eccParams,keyPair.pub,data,signature);
if (!verified) {
fail("verification failed!");
}
As you can see, creating and verifying ECC signatures can be a simple process. Contact us for a commercial license.